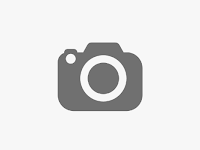
Tutorial Exception Handling SAP ABAP
Exception is a problem that arises during the execution of a program. If an exception occurs, the normal program flow is interrupted and the application program terminates abnormally, so the exception must be handled immediately.
Exceptions provide a way to transfer control from one part of the program to another. In SAP ABAP exception handling can be resolved with 3 keywords, RAISE, TRY, CATCH and CLEANUP. Assuming a block will raise an exception, a method of catching exceptions uses a combination of the TRY and CATCH keywords. The TRY - CATCH block is placed around code that might produce an exception. Following is the syntax for using TRY - CATCH -
TRY.
TRY.
Try Block <Code that raises an exception>
CATCH
Catch Block <exception handler M>
. . .
. . .
. . .
CATCH
Catch Block <exception handler R>
CLEANUP.
Cleanup block <to restore consistent state>
ENDTRY.
RAISE - Exception is proposed to show that some extraordinary situations have occurred. Usually, an exception handler tries to correct an error or find an alternative solution.TRY − TRY block contains the encoding for the application whose exception will be handled. These block statements will be processed sequentially. Can contain further control structures and procedure calls or other ABAP programs. This is followed by one or more CATCH blocks.
CATCH − the program catches an exception with an exception handler in the place in the program where you want to handle this problem. The CATCH keyword indicates catching an exception.
CLEANUP - The CLEANUP statement is run when an exception occurs in the TRY block that is not captured by the same TRY - ENDTRY handler. In the CLEANUP clause, the system can return objects to a consistent state or release external resources. That is, cleaning work can be done for the TRY block context.
Read too: Tutorial Dasar SAP ABAP
Following is the Attribute of the exception:
No | Attribute & Description |
---|---|
1 | Textid Used to define different text for exceptions and also affect the results of the get_text method.. |
2 | Previous This attribute can save original exceptions that allow you to build chains of exceptions. |
3 | get_text returns textual representations as strings according to the exception system language |
4 | get_longtext returns the old variant of the exception textual representation as a string. |
5 | get_source_position Provides the name of the program and the line number reached where the exception was raised successfully |
Contoh
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
| REPORT ZExceptionsDemo. PARAMETERS Num_1 TYPE I. DATA res_1 TYPE P DECIMALS 2. DATA orf_1 TYPE REF TO CX_ROOT. DATA txt_1 TYPE STRING. start - of - selection. Write: / 'Square Root and Division with:' , Num_1. write: / . TRY. IF ABS ( Num_1 ) > 150. RAISE EXCEPTION TYPE CX_DEMO_ABS_TOO_LARGE. ENDIF. TRY. res_1 = SQRT( Num_1 ). Write: / 'Result of square root:' , res_1. res_1 = 1 / Num_1. Write: / 'Result of division:' , res_1. CATCH CX_SY_ZERODIVIDE INTO orf_1. txt_1 = orf_1?GET_TEXT( ). CLEANUP. CLEAR res_1. ENDTRY. CATCH CX_SY_ARITHMETIC_ERROR INTO orf_1. txt_1 = orf_1?GET_TEXT( ). CATCH CX_ROOT INTO orf_1. txt_1 = orf_1?GET_TEXT( ). ENDTRY. IF NOT txt_1 IS INITIAL. Write / txt_1. ENDIF. Write: / 'Final Result is:' , res_1. |
Square Root and Division with: 160
The absolute value of a number is too high
Final Result is: 0.00
In the example above, if the number is greater than 150, the exception CX_DEMO_ABS_TOO_LARGE diraised.